This text is a write-up of a chat I gave at MinneBar 2022. As an alternative of studying this, you possibly can additionally watch the recording or view the slides.
The title of this speak is “sustaining software program correctness.” However what precisely do I imply by “correctness”? Let me set the scene with an instance.
Years in the past, when Trello Android adopted RxJava, we additionally adopted a reminiscence leak downside. Earlier than RxJava, we’d have, say, a button and a click on listener; when that button would go away so would its click on listener. However with RxJava, we now have a button click on stream and a subscription, and that subscription might leak reminiscence.
We might keep away from the leak by unsubscribing from every subscription, however manually managing all these subscriptions was a ache, so I wrote RxLifecycle to deal with that for me. I’ve since disavowed RxLifecycle because of its quite a few shortcomings, one among which was that you simply needed to bear in mind to use it accurately to each subscription:
observable
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.bindToLifecycle() // Neglect this and leak reminiscence!
.subscribe()
In the event you put bindToLifecycle()
earlier than subscribeOn()
and observeOn()
it’d fail. Furthermore, for those who outright neglect so as to add bindToLifecycle()
it doesn’t work, both!
There have been a whole bunch (maybe 1000’s) of subscriptions in our codebase. Did everybody bear in mind so as to add that line of code each time, and in the correct place? No, after all not! Folks forgot consistently, and whereas code assessment caught it generally, it didn’t at all times, resulting in reminiscence leaks.
It’s simple responsible folks for messing this up, however actually the design of RxLifecycle itself was at fault. Relying on folks to “simply do it proper” will ultimately fail.
Let’s generalize this story.
Suppose you’ve simply created a brand new structure, library, or course of. Over time you discover some points that stem from folks incorrectly utilizing your creation. If folks would simply use every thing accurately there wouldn’t be any issues, however to your horror everybody continues to make errors and trigger your software program to fail.
That is what I name the correctness dilemma: it’s simple to create however arduous to take care of. Getting folks to align on a code type, correctly contribute to an OSS venture, or constantly releasing good builds – all of those processes are simple to give you, however errors ultimately creep in when folks do not use them accurately.
The core mistake is designing with out maintaining human fallibility in thoughts. Anticipating folks to be good just isn’t a tenable answer.
In the event you pay no consideration to this side of software program design (like I did for a lot of my profession), you might be setting your self up for long run failure. Nevertheless, as soon as I began specializing in this downside, I found many good (and customarily simple) options. All it’s important to do is strive, just a bit bit, and generally you’ll arrange a product that lasts eternally.
How can we design for correctness?
Human error is an issue in any business, however I feel that within the software program business we have now a singular superpower that lets us sidestep this downside: we will readily flip human processes into software program processes. We are able to take unreliable chores completed by folks and switch them into reliable code, and quicker than anybody else as a result of we’ve bought all of the software program builders.
What can we do with this energy to keep away from human fallibility? We constrain. The important thing concept is that the much less freedom you give, the extra doubtless you’ll keep correctness. If in case you have the liberty to do something, then you may have the liberty to make each mistake. In the event you’re constrained to solely do the right factor, then you haven’t any alternative however to do the correct factor!
There are all types of methods we will make use of for correctness, laying on a spectrum between flexibility and rigidity:
Let’s take a look at every technique in flip.
Institutional Data
In any other case referred to as “stuff in your head.”
That is much less of a method and extra of a place to begin. All the things has to begin someplace, and often that’s within the collective consciousness of you and your teammates.
Ideas are nice! Pondering comes naturally to most individuals and have many benefits:
Ideas are extraordinarily low-cost; the going price has been unaffected by inflation, so it’s nonetheless only a penny for a thought. Brainstorming is predicated on how low-cost ideas are; “The place ought to this button go?” you may ask, and also you’ll have fifteen totally different attainable places within the span of some minutes.
Ideas are extraordinarily versatile. You’ll be able to pitch a brand new course of to your crew to check out for per week, see the way it goes, then abandon it if it fails. “Let’s strive posting a fast standing message every morning”, you may counsel, and when everybody inevitably hates it then you’ll be able to rapidly give it up per week later.
Institutional information can clarify and summarize code. Would you slightly learn by way of each line of code, or have somebody focus on its construction and objectives? Trello Android might function offline, which implies writing modifications to the consumer’s database then syncing these modifications with the server – I’ve simply now described tens of 1000’s of strains of code in a single sentence.
Institutional information can clarify the “why” of issues. By itself, code can solely describe the way it will get issues completed, however not why. Any hack you write to unravel an answer in a roundabout means ought to embrace a touch upon why the hack was essential, lest future generations surprise why you wrote such wacky code. There might need been a collection of experiments that decided that is the most effective answer, regardless that that’s not apparent.
Institutional information can describe human issues. There’s solely a lot you are able to do with code. Your trip coverage can’t be totally encoded as a result of staff get to decide on once they take trip, not computer systems!
There’s so much to love about considering, however in terms of correctness, institutional information is the worst. Low cost and versatile doesn’t make for a robust correctness basis:
Institutional information will be misremembered, forgotten, or go away the corporate. I are inclined to neglect most issues I did after only a few months. Coworkers with skilled information can stop anytime they need.
Institutional information is laborious to share. Each new teammate must be taught each little bit of institutional information by another person throughout onboarding. Everytime you give you a brand new concept, it’s important to talk it to each current teammate, too. Scale is unimaginable.
Institutional information will be troublesome to speak. The sport “phone” is based on simply how arduous it’s to move alongside easy messages. Now think about taking part in phone with some troublesome technical idea.
Institutional information doesn’t remind folks to do one thing. Do you want somebody to press a button each week to deploy the newest construct to manufacturing? What if the one who does it… simply forgets? What in the event that they’re on trip and nobody else remembers that somebody has to push the button?
Like I stated, institutional information is sweet and necessary – it’s the place to begin, and an affordable, versatile method to experiment. However any institutional information that’s repeatedly used must be codified indirectly. Which leads us to…
Documentation
I’m certain that somebody was screaming at their monitor whereas studying the final part being like “Documentation! Duh! That’s the reply!”
Documentation is institutional information that’s written down. That makes it tougher to neglect and simpler to transmit.
Documentation has a lot of the benefits of institutional information – although not fairly as low-cost or versatile, it’s also in a position to summarize code and describe human issues. Additionally it is a lot simpler to broadcast documentation; you don’t have to sit down down and have a dialog with each one who must be taught.
There’s additionally a pair bonuses to visible information. Documentation can use footage or video. An excellent movement chart or structure abstract is value 1000 phrases – I might spend a bunch of time speaking about how Trello Android’s offline structure works, or you possibly can take a look at the movement charts on this article. I personally discover that video can click on with me simpler than simply speaking; I believe because of this the trendy video essay exists (over written articles).
Documentation also can create checklists for advanced processes. We automated a lot of it, however the technique of releasing a brand new model of Trello Android nonetheless concerned many unavoidably handbook steps (e.g. writing launch notes or checking crash stories for brand new points). An excellent guidelines will help minimize down on human error.
Regardless of documentation’s advantages, there’s a cause this speak was initially titled “documentation just isn’t sufficient.”
Right here’s a typical scenario we’d run into at work: we’d give you a brand new crew course of or structure, and folks would say “that is nice, however we’ve bought to put in writing it down so folks gained’t make errors sooner or later.” We’d take the time to put in writing some nice documentation… solely to find that errors stored occurring. What offers?
Properly, it seems there are lots of issues that may come up with documentation:
Documentation will be badly written or misunderstood. A doc can clarify an idea poorly or inaccurately, or the reader may merely misapprehend its that means. There’s additionally no method to double-check that the data was transmitted successfully; speaking to a different individual permits for clarifying questions, however studying documentation is a one-way transmission.
Documentation will be poorly maintained and go outdated. Maybe your doc was correct when first written, however years later, it’s a web page of lies. Protecting documentation up-to-date is dear and laborious, for those who even bear in mind to return and replace it.
Documentation will be arduous to search out or just ignored. Even when the doc is ideal, you want to have the ability to discover it! Possibly it’s someplace on Confluence however who is aware of the place. Even worse, folks may not even know they should learn some documentation! “I’m sorry I took down the server, I didn’t know that you simply could not minimize releases at 11PM as a result of I by no means noticed the discharge course of doc.”
Documentation can’t function a reminder. Very like with institutional information, there’s no means for documentation to inform you to do one thing at a sure time. Checklists get you barely nearer, however there’s no assure that an individual will bear in mind to examine the guidelines! Trello Android had a launch guidelines, however oftentimes the discharge would roll round and we’d uncover that somebody forgot to examine it, and now we will’t translate the discharge notes in time.
Documentation is critical. Some ideas can solely be documented, not codified (like high-level structure explanations). And in the end, software program growth is about working with people. People are messy, and solely written language can deal with that messiness. Nevertheless, it’s just one step above institutional information by way of correctness.
Affordances
Let’s take a detour into the dictionary.
An affordance is “the standard or property of an object that defines its attainable makes use of or makes clear the way it can or must be used.”
I used to be first launched to this idea by “The Design of On a regular basis Issues” by Don Norman, which matches into element finding out seemingly banal design decisions which have enormous impacts on utilization.
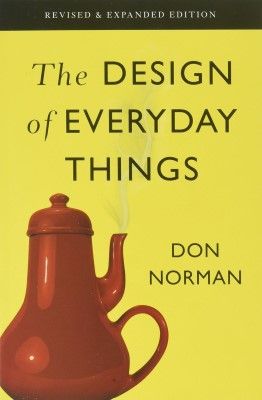
A traditional instance of excellent and unhealthy affordances are doorways. Good doorways have an apparent method to open them. Crash bar doorways are instance of that; there’s no universe during which you’d assume to pull these doorways open.
The alternative is what is called a Norman door (named after the aforementioned Don Norman). Norman doorways that invite you to do the flawed factor, for instance by having a deal with that begs to be pulled however, the truth is, must be pushed.
Right here’s why I discover all this attention-grabbing: We are able to use affordances in software program to invisibly information folks in direction of correctness in software program. In the event you make “doing the correct factor” pure, folks will simply do it with out even realizing they’re being guided.
Right here’s an instance of an affordant API: in Android, there’s nobody stopping you from opening a connection to a database everytime you need. A dozen builders every doing their very own customized DB transactions could be a nightmare, so as a substitute, on Trello Android we added a “modification” API that might replace the DB on request. The modification API was simple – you’ll simply say “create a card” and it’d go do it. That’s so much easier than opening your personal connection, organising a SQL question, and committing it – thus we by no means needed to fear about anybody doing it manually. Why would you, when utilizing the modification API was there?
What about enhancing non-software conditions? One instance that involves thoughts is submitting bug stories. The tougher it’s to file a bug report, the much less doubtless you might be to get one (which, hey, possibly that’s a function for you, however not for me). The teams that put the onus on the filer to determine precisely the place and the way to file a bug tended to not hear necessary suggestions, whereas the groups that stated “we settle for all bugs, we’ll filter out what’s not necessary” bought numerous suggestions on a regular basis.
If, for some cause, you’ll be able to’t make the “proper” means of doing issues any extra affordant, you’ll be able to as a substitute do the alternative and make the flawed means un-affordant (aka arduous and obtuse). Is there an escape hatch API that most individuals shouldn’t use? Disguise it in order that solely those that want it will probably even discover it. Getting too many developer job functions? Add a easy algorithm filter to the beginning of your interview pipeline.
I consider this idea like how governments can form financial coverage by way of subsidies and taxes: make what you need folks to do low-cost; make what you don’t need folks to do costly.
Although not precisely an affordance, I additionally contemplate peer stress a associated method to invisibly nudge folks in the correct course. I don’t assume I’m alone once I say that the very first thing I do in a codebase is go searching and attempt to copy the native type and logic. If somebody asks me so as to add a button that makes a community request, I’m going to search out one other button that does it first, copy and paste, then edit. If there are 50 other ways to put in writing that code, effectively, I hope I discovered the correct one to repeat; if there’s only one, then I’m going to repeat the write technique. Consistency creates a flywheel for itself.
I really like affordances as a result of they information folks with out them being consciously conscious of it. A variety of the correctness methods I’ll focus on later are extra heavy handed and obtrusive; affordances are light and invisible.
Their foremost draw back is that affordances and peer stress can solely information, not prohibit. Usually these methods are helpful while you can’t cease somebody from doing the flawed factor as a result of the coding language/framework is just too permissive, you might want to present exceptions for uncommon circumstances, otherwise you’re coping with human processes (and something can go off the rails there).
Software program Checks
Software program checks are when code can examine itself for correctness.
In the event you’re something like me, you’ve simply began skimming this part since you assume I’m gonna be speaking about unit assessments. Properly… okay, sure, I’m, however software program checks are a lot extra than unit assessments. Unit assessments are only one type of a software program examine, however there are lots of others, such because the compiler checking grammar.
What pursuits me right here is the timing of every software program examine. These checks can occur as early as while you’re writing code to as late while you’re operating the app.
The sooner you will get suggestions, the higher. Quick suggestions creates a decent loop – you neglect a semicolon, the IDE warns you, you repair it earlier than even compiling. Against this, gradual suggestions is painful – you’ve simply launched the newest model of your app and oops, it’s crashing for 25% of customers, it’ll be at the least a day earlier than you’ll be able to roll out a repair, and also you’ll need to undo some structure decisions alongside the best way.
Let’s take a look at the timing of software program checks, from slowest to quickest:
The slowest software program examine is a runtime examine, whereby you examine for correctness as this system is operating. Gathering analytics/crash information out of your software program because it runs is sweet for locating issues. For instance, in OkHttp, every Name
can solely be used as soon as; attempt to reuse it and also you get an exception. This examine is unimaginable to make earlier than operating the software program.
There are massive drawbacks to runtime checks: your customers find yourself being your testers (which gained’t make them comfortable) and there’s a protracted turnaround from discovering an issue to deploying a repair (which additionally gained’t make your customers comfortable). It’s additionally an inconsistent method to check your code – there is likely to be a bug on a code path that’s solely accessed as soon as a month, making the suggestions loop even slower. Runtime checks are value embracing as a final resort, however counting on them alone is poor follow.
The subsequent slowest software program examine is a handbook check, the place you manually execute code that runs a examine. These will be unit assessments, integration assessments, regression assessments, and many others. There will be quite a lot of worth in writing these assessments, however it’s important to foster a tradition for testing (because it takes time & effort to put in writing and confirm the correctness of assessments). I feel it’s value investing in these types of assessments; in the long term, good assessments not solely prevent effort but in addition drive you to architect your code in (what I contemplate) a usually superior means.
One step up from handbook assessments are automated assessments, that are simply handbook assessments that run routinely. The core downside with handbook assessments is that it requires somebody to recollect to run them. Why not make a pc bear in mind to do it as a substitute? Bonus factors if failed checks stop one thing unhealthy from occurring (e.g. blocking code merges that break the construct).
Subsequent up are compile time checks, whereby the compilation step checks for errors. Sometimes that is in regards to the compiler implementing its personal guidelines, akin to static kind security, however you’ll be able to combine a lot extra into this step. You’ll be able to have checks for code type, linting, protection, and even run some automated assessments throughout compilation.
Lastly, the quickest suggestions is given at design time, the place your editor itself tells you that you simply made a mistake when you are writing code. As an alternative of discovering out you mis-named a variable throughout compilation, the editor can immediately inform you that there’s a typo. Or while you’re writing an article, the spellchecker can discover errors earlier than you submit the article on-line. Very like compile time checks, whereas these are typically about grammatical errors, you’ll be able to generally insert your personal design time type/lint/and many others. checks.
Whereas quick suggestions is healthier, the quicker timings are inclined to constrain what you’ll be able to check. Design-time checks can solely particular bits of logic, whereas runtime checks can cowl mainly something your software program can do. In my expertise, whereas it’s simpler to implement runtime checks, it’s usually value placing in a bit of additional effort to make these checks go quicker (and be run extra constantly).
Constraints
Constraints make it in order that the one path is the right one, such that it’s unimaginable to do the flawed factor. Let’s take a look at a number of circumstances:
Enums vs. strings. In the event you can constrain to only a few choices (as a substitute of any string) it makes your life simpler. For instance, persons are usually tempted to make use of stringly-typing when deciphering information from server APIs (e.g. “card”, “board”, “checklist”). However strings will be something, together with information that your software program just isn’t in a position to deal with. By utilizing an enum as a substitute (CARD
, BOARD
, LIST
) you’ll be able to constrain the remainder of your software to only the legitimate choices.
Stateless capabilities vs. stateful lessons. Something with state runs the danger of ending up in a foul state, the place two variables are in stark disagreement with one another. In the event you can execute the identical logic in a self-contained, stateless operate, there’s no danger that some long-lived variables can find yourself out of alignment with one another.
Pull requests vs. merging to foremost. In the event you let anybody merge code to foremost, then you definitely’ll find yourself with failing assessments and damaged builds. By requiring folks to undergo a pull request – thus permitting steady integration to run – you’ll be able to drive higher habits in your codebase.
Not solely can constraints assure correctness, additionally they restrict the logical headspace you might want to wrap your thoughts round a subject. As an alternative of needing to contemplate each string, you’ll be able to contemplate a restricted variety of enums. In the identical vein, it additionally limits the variety of assessments you might want to cowl your logic.
Automation
If you automate, a pc does every thing for you. This is sort of a constraint however higher as a result of folks don’t even need to do something. You solely have to put in writing the automation as soon as, then the computer systems will take over doing all your busywork.
One efficient use of this technique is code era. A traditional instance are Java POJOs, which don’t include an equals()
, hashCode()
, or toString()
implementations. Within the outdated days, you used to need to generate these by hand; these implementations would rapidly go stale as you modified the POJO’s fields. Now, we have now libraries like AutoValue (which generate implementations primarily based on annotations) or languages like Kotlin (which generate implementations as a language function).
Steady integration is one other nice automation technique. Having hassle remembering to run all of your checks earlier than merging new code? Simply get CI to drive you to do it by not permitting a merge till you move all of the assessments. You’ll be able to even have CI do automated deployments, such that you simply barely need to do something after merging code earlier than releasing it.
There are two foremost drawbacks of automation. The primary is that it’s costly to put in writing and keep, so it’s important to examine that the payoff is value the fee. The second downside is that automation can do the flawed factor over and over, so it’s important to watch out to examine that you simply carried out the automation accurately within the first place.
Now that we’ve reviewed the methods, enable me to reveal how we use them in the actual world.
Earlier than fixing any given downside, you must take a step again and determine which of those methods to use (if any) earlier than committing to an answer. You’ll in all probability find yourself with a mixture of methods, not only one. For instance, it’s not often the case that you could simply implement constraints or automation with out additionally documenting what you probably did.
There are a number of meta-considerations to consider as effectively:
First, whereas inflexible options (like constraints or automation) are higher for correctness, they’re worse for flexibility. They’re costly to vary after implementation and unforgiving of exceptions. Thus, you might want to steadiness correctness and suppleness for every scenario. Basically, I pattern in direction of early flexibility, then transferring in direction of correctness as essential.
Second, you may implement correctness badly. You’ll be able to have flakey software program checks, overbearing code contribution processes, troublesome automation upkeep, or no escape hatches for brand new options or exceptions. Correctness is an funding, and you might want to be sure to can afford to speculate and keep.
Final, you want buy-in out of your teammates. I are inclined to make the error of considering that as a result of I like an answer that everybody else will even prefer it, however that’s undoubtedly not at all times the case. In the event you get settlement from others, correctness is less complicated to implement (particularly for crew processes); folks will associate with your plans, and even pitch in concepts to enhance it.
Disagreements, alternatively, can result in toxicity, akin to folks ignoring or purposefully undermining your creation. At my first job they tried to implement a code type checker that prevented merges, however did not have a plan for the way to repair outdated recordsdata. There was no automated formatter (as a result of it was a customized markup language), so nobody ever wished to repair the large recordsdata; as a substitute everybody simply stored utilizing a workaround to keep away from the code type checker! Whoops!
Taking a while to assemble proof then presenting the case to your coworkers could make a world of distinction.
Now, let’s take a look at a number of examples and analyze them…
Code Fashion
For instance, how do you get everybody to constantly use areas over tabs?
❌ Institutional information – Unhealthy; this doesn’t stop folks from going off the code type in any respect.
❌ Documentation – Simply as unhealthy as institutional information, however written down.
✅ Affordances – Semi-effective. You’ll be able to configure your editor to at all times use areas as a substitute of tabs. Even higher, some IDEs allow you to examine a code type definition into supply management so everyone seems to be on the identical web page style-wise. Nevertheless, by way of correctness, it guides however doesn’t prohibit.
✅ Software program checks – Utilizing lint or code type checkers to confirm code type is a good use of CPU cycles. Folks can’t merge code that goes off type with this in place.
❌ Constraints – Not likely attainable from what I can inform. I’m unsure the way you’d implement this – ship everybody keyboards with out the tab key?
❌ Automation – You possibly can have some hook routinely rewrite tabs to areas, however truthfully this offers me the heebie jeebies a bit!
In the long run, I like implementing your type with software program checks, however making it simpler to keep away from failures with affordances.
Code Contribution to an OSS Venture
How do folks contribute code to an open supply codebase? In the event you’ve bought a selected course of (like code critiques, operating assessments, deploying) how do you guarantee these occur when a random individual donates code?
❌ Institutional information – Inconceivable for strangers.
✅ Documentation – In the event you write strong directions, you’ll be able to create a extra welcoming atmosphere for anybody to contribute code. Nevertheless, documentation alone won’t end in a dependable course of, as a result of not everybody reads the handbook.
✅ Affordances – There’s lots you are able to do right here, like templates for explaining your code contribution, or giving folks clear buttons for various contributor actions (like signing the contributor license settlement).
✅ Software program checks – Having loads of software program checks in place makes it a lot simpler for folks to contribute code that doesn’t break the prevailing venture.
✅ Constraints – Repository hosts allow you to put all types of good constraints on code contribution: stop merging on to foremost, require code critiques, require contributor licenses, require CI to move earlier than merging.
✅ Automation – CI is critical as a result of it feeds data into the constraints you’ve arrange.
For this, I take advantage of a mixture of all totally different methods to attempt to get folks to do the correct factor.
Cleansing Streams
Let’s revisit the story from the start of this text – the way to clear up assets in reactive streams of knowledge (particularly with RxJava).
❌ Institutional information – You’ll be able to educate folks to wash up streams, however they may neglect.
❌ Documentation – No extra appropriate than institutional information, simply simpler to unfold the data.
✅ Affordances – We used an RxJava software known as CompositeDisposable
to wash up a bunch of streams without delay. AutoDispose provides simpler methods to wash up streams routinely as effectively. Nevertheless, all these options nonetheless require remembering to make use of them.
✅ Software program checks – We added RxLint to confirm that we truly deal with the returned stream subscription. Nevertheless, this doesn’t assure you keep away from a leak, simply that you simply made an try and keep away from it. In the event you’re utilizing AutoDispose, it offers a lint examine to ensure it’s getting used.
✅ Constraints – I’m fairly excited by Kotlin coroutines’ scopes right here. As an alternative of placing the onus on the developer to recollect to wash up, a coroutine scope requires that you simply outline the lifespan of the coroutine.
❌ Automation – Realizing when a stream of knowledge is now not wanted is one thing solely people can decide.
What technique you employ right here is dependent upon the library. One of the best answer IMO are constraints, the place the library itself forces you to keep away from leaks. In the event you’re utilizing a library that may’t implement it (like RxJava), then affordances and software program checks are the best way to go.
Clearly, not each possibility is accessible to each downside – you’ll be able to’t automate your means out of all software program growth! Nevertheless, at its core, the much less folks need to make decisions, the higher for correctness. Free folks’s minds up for what actually issues – growing software program, slightly than wrestling with avoidable errors.