Within the earlier article of this Python collection, we shared a short introduction to Python, its command-line shell, and the IDLE.
We additionally demonstrated tips on how to carry out arithmetic calculations, tips on how to retailer values in variables, and tips on how to print again these values to the display. Lastly, we defined the ideas of strategies and properties within the context of Object Oriented Programming by way of a sensible instance.
On this information, we are going to focus on management movement (to decide on completely different programs of motion relying on the knowledge entered by a consumer, the results of a calculation, or the present worth of a variable) and loops (to automate repetitive duties) after which apply what we have now discovered to this point to jot down a easy shell script that may show the working system kind, the hostname, the kernel launch, model, and the machine {hardware} identify.
This instance, though primary, will assist us illustrate how we are able to leverage Python OOP’s capabilities to jot down shell scripts simpler than utilizing common bash instruments.
In different phrases, we wish to go from
# uname -snrvm

to

or

Seems fairly, doesn’t it? Let’s roll up our sleeves and make it occur.
Be taught Management Movement in Python
As we stated earlier, management movement permits us to decide on completely different outcomes relying on a given situation. Its most straightforward implementation in Python is an if / else clause.
The essential syntax is:
if situation: # motion 1 else: # motion 2
- When the situation evaluates to true, the code block beneath will likely be executed (represented by
# motion 1
. In any other case, the code underneath else will likely be run. - A situation will be any assertion that may consider as both true or false.
For instance:
1 < 3 # true firstName == "Gabriel" # true for me, false for anybody not named Gabriel
- Within the first instance, we in contrast two values to find out if one is bigger than the opposite.
- Within the second instance we in contrast firstName (a variable) to find out if, on the present execution level, its worth is similar to “Gabriel”
- The situation and the else assertion have to be adopted by a colon (:)
- Indentation is essential in Python. Strains with similar indentation are thought of to be in the identical code block.
Please observe that the if / else assertion is just one of many many management movement instruments out there in Python. We reviewed it right here since we are going to use it in our script later. You’ll be able to study extra about the remainder of the instruments within the official docs.
Be taught Loops in Python
Merely put, a loop is a sequence of directions or statements which can be executed so as so long as a situation is true, or as soon as per merchandise in an inventory.
The simplest loop in Python is represented by the for loop that iterates over the objects of a given checklist or a string starting with the primary merchandise and ending with the final.
Primary syntax:
for x in instance: # do that
Right here instance will be both an inventory or a string. If the previous, the variable named x represents every merchandise within the checklist; if the latter, x represents every character within the string:
>>> rockBands = [] >>> rockBands.append("Roxette") >>> rockBands.append("Weapons N' Roses") >>> rockBands.append("U2") >>> for x in rockBands: print(x) or >>> firstName = "Gabriel" >>> for x in firstName: print(x)
The output of the above examples is proven within the following picture:
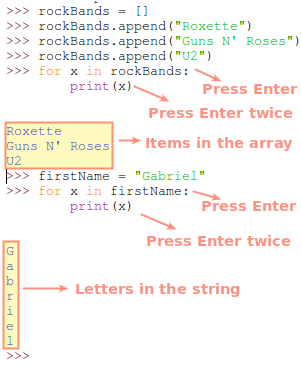
Python Modules
For apparent causes, there have to be a approach to save a sequence of Python directions and statements in a file that may be invoked when it’s wanted.
That’s exactly what a module is. Notably, the os module offers an interface to the underlying working system and permits us to carry out lots of the operations we often do in a command-line immediate.
As such, it incorporates a number of strategies and properties that may be referred to as as we defined within the earlier article. Nonetheless, we have to import (or embody) it in our surroundings utilizing the import key phrase:
>>> import os
Let’s print the present working listing:
>>> os.getcwd()
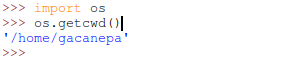
Let’s now put all of this collectively (together with the ideas mentioned within the earlier article) to jot down the specified script.
Python Script
It’s thought of good follow to start out a script with an announcement that signifies the aim of the script, the license phrases underneath which it’s launched, and a revision historical past itemizing the adjustments which were made. Though that is extra of a private choice, it provides an expert contact to our work.
Right here’s the script that produces the output we have now proven on the high of this text. It’s closely commented on so to perceive what’s occurring.
Take a couple of minutes to undergo it earlier than continuing. Notice how we use an if / else construction to find out whether or not the size of every discipline caption is bigger than the worth of the sphere itself.
Primarily based on the outcome, we use empty characters to fill within the area between a discipline caption and the subsequent. Additionally, we use the best variety of dashes as a separator between the sphere caption and its worth beneath.
#!/usr/bin/python3 # Change the above line to #!/usr/bin/python if you do not have Python 3 put in # Script identify: uname.py # Function: Illustrate Python's OOP capabilities to jot down shell scripts extra simply # License: GPL v3 (http://www.gnu.org/licenses/gpl.html) # Copyright (C) 2016 Gabriel Alejandro Cánepa # Fb / Skype / G+ / Twitter / Github: gacanepa # Electronic mail: gacanepa (at) gmail (dot) com # This program is free software program: you may redistribute it and/or modify # it's underneath the phrases of the GNU Basic Public License as printed by # the Free Software program Basis, both model 3 of the License, or # (at your possibility) any later model. # This program is distributed within the hope that will probably be helpful, # however WITHOUT ANY WARRANTY; with out even the implied guarantee of # MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the # GNU Basic Public License for extra particulars. # You need to have obtained a duplicate of the GNU Basic Public License # together with this program. If not, see <http://www.gnu.org/licenses/>. # REVISION HISTORY # DATE VERSION AUTHOR CHANGE DESCRIPTION # ---------- ------- -------------- # 2016-05-28 1.0 Gabriel Cánepa Preliminary model # Import the os module import os # Assign the output of os.uname() to the the systemInfo variable # os.uname() returns a 5-string tuple (sysname, nodename, launch, model, machine) # Documentation: https://docs.python.org/3.2/library/os.html#module-os systemInfo = os.uname() # It is a mounted array with the specified captions within the script output headers = ["Operating system","Hostname","Release","Version","Machine"] # Preliminary worth of the index variable. It's used to outline the # index of each systemInfo and headers in every step of the iteration. index = 0 # Preliminary worth of the caption variable. caption = "" # Preliminary worth of the values variable values = "" # Preliminary worth of the separators variable separators = "" # Begin of the loop for merchandise in systemInfo: if len(merchandise) < len(headers[index]): # A string containing dashes to the size of merchandise[index] or headers[index] # To repeat a personality(s), enclose it inside quotes adopted # by the star signal (*) and the specified variety of instances. separators = separators + "-" * len(headers[index]) + " " caption = caption + headers[index] + " " values = values + systemInfo[index] + " " * (len(headers[index]) - len(merchandise)) + " " else: separators = separators + "-" * len(merchandise) + " " caption = caption + headers[index] + " " * (len(merchandise) - len(headers[index]) + 1) values = values + merchandise + " " # Increment the worth of index by 1 index = index + 1 # Finish of the loop # Print the variable named caption transformed to uppercase print(caption.higher()) # Print separators print(separators) # Print values (objects in systemInfo) print(values) # INSTRUCTIONS: # 1) Save the script as uname.py (or one other identify of your selecting) and provides it execute permissions: # chmod +x uname.py # 2) Execute it: # ./uname.py
After getting saved the above script to a file, give it execute permissions and run it as indicated on the backside of the code:
# chmod +x uname.py # ./uname.py
If you happen to get the next error whereas making an attempt to execute the script:
-bash: ./uname.py: /usr/bin/python3: unhealthy interpreter: No such file or listing
It means you don’t have Python 3 put in. If that’s the case, you may both set up the bundle or substitute the interpreter line (pay particular consideration and be very cautious when you adopted the steps to replace the symbolic hyperlinks to the Python binaries as outlined within the earlier article):
#!/usr/bin/python3
with
#!/usr/bin/python
which is able to trigger the put in model of Python 2 to execute the script as an alternative.
Notice: This script has been examined efficiently each in Python 2.x and 3.x.
Though considerably rudimentary, you may consider this script as a Python module. This implies that you may open it within the IDLE (File → Open… → Choose file):
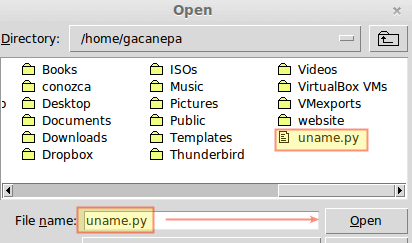
A brand new window will open with the contents of the file. Then go to Run → Run module (or simply press F5). The output of the script will likely be proven within the unique shell:
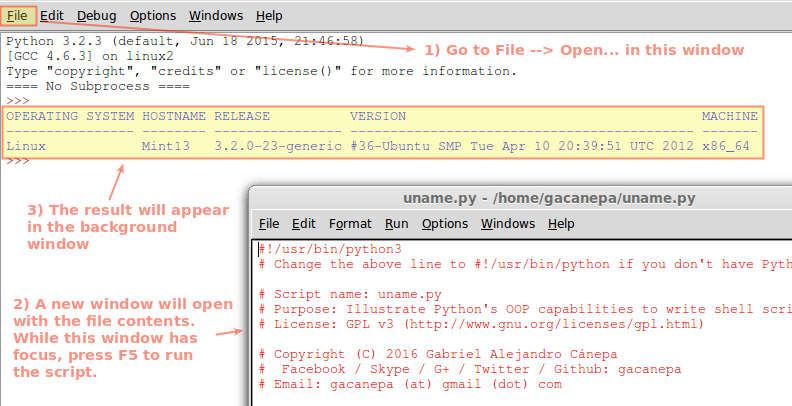
If you wish to acquire the identical outcomes with a script written purely in Bash, you would wish to make use of a mixture of awk, sed, and resort to complicated strategies to retailer and retrieve objects in an inventory (to not point out the usage of tr to transform lowercase letters to uppercase).
As well as, Python offers portability in that every one Linux techniques ship with a minimum of one Python model (both 2.x or 3.x, generally each). Ought to that you must depend on a shell to perform the identical objective, you would wish to jot down completely different variations of the script primarily based on the shell.
This goes to indicate that Object Oriented Programming options can turn out to be robust allies of system directors.
Notice: You’ll find this python script (and others) in one in all my GitHub repositories.
Abstract
On this article, we have now reviewed the ideas of management movement, loops/iteration, and modules in Python. We’ve proven tips on how to leverage OOP strategies and properties in Python to simplify in any other case complicated shell scripts.
Do you’ve gotten every other concepts you wish to check? Go forward and write your individual Python scripts and tell us when you have any questions. Don’t hesitate to drop us a line utilizing the remark type beneath, and we are going to get again to you as quickly as we are able to.