It has been stated (and sometimes required by recruitment businesses) that system directors have to be proficient in a scripting language. Whereas most of us could also be comfy utilizing Bash (or different Linux shells of our selection) to run command-line scripts, a robust language equivalent to Python can add a number of advantages.
To start with, Python permits us to entry the instruments of the command-line setting and to utilize Object Oriented Programming options (extra on this later on this article).
On high of it, studying Python can enhance your profession within the fields of creating desktop functions and studying information science.
Being really easy to be taught, so vastly used, and having a plethora of ready-to-use modules (exterior information that include Python statements), no surprise Python is the popular language to show programming to first-year pc science college students in the US.
On this 2-article sequence, we are going to evaluation the basics of Python in hopes that one can find it helpful as a springboard to get you began with programming and as a fast reference information afterward.
That stated, let’s get began.
Set up Python on Linux
Python variations 2.x and 3.x are often out there in most fashionable Linux distributions out of the field. You may enter a Python shell by typing python
or python3
in your terminal emulator and exit with give up()
:
$ which python $ which python3 $ python -v $ python3 -v $ python >>> give up() $ python3 >>> give up()
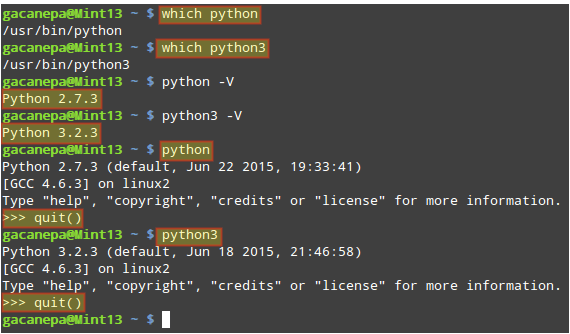
If you wish to discard Python 2.x and use 3.x as a substitute if you kind python, you possibly can modify the corresponding symbolic hyperlinks as follows:
$ sudo rm /usr/bin/python $ cd /usr/bin $ ln -s python3.2 python # Select the Python 3.x binary right here
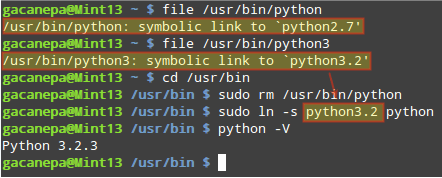
By the best way, you will need to word that though variations 2.x are nonetheless used, they don’t seem to be actively maintained. For that motive, chances are you’ll need to take into account switching to 3.x as indicated above. Since there are some syntax variations between 2.x and 3.x, we are going to concentrate on the latter on this sequence.
To put in Python 3.x in your respective Linux distributions, run:
$ sudo apt set up python3 [On Debian, Ubuntu and Mint] $ sudo yum set up python3 [On RHEL/CentOS/Fedora and Rocky/AlmaLinux] $ sudo emerge -a dev-lang/python [On Gentoo Linux] $ sudo apk add python3 [On Alpine Linux] $ sudo pacman -S python3 [On Arch Linux] $ sudo zypper set up python3 [On OpenSUSE]
Set up Python IDLE on Linux
One other manner you should utilize Python in Linux is thru the IDLE (the Python Built-in Improvement Surroundings), a graphical person interface for writing Python code.
$ sudo apt set up idle [On Debian, Ubuntu and Mint] $ sudo yum set up idle [On RHEL/CentOS/Fedora and Rocky/AlmaLinux] $ sudo apk add idle [On Alpine Linux] $ sudo pacman -S idle [On Arch Linux] $ sudo zypper set up idle [On OpenSUSE]
As soon as put in, you will note the next display screen after launching the IDLE. Whereas it resembles the Python shell, you are able to do extra with the IDLE than with the shell.
For instance, you possibly can:
1. open exterior information simply (File → Open).
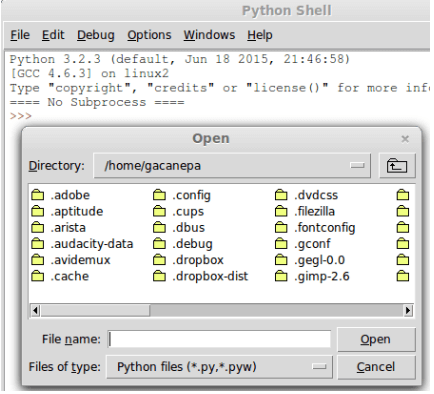
2) copy (Ctrl + C)
and paste (Ctrl + V)
textual content, 3) discover and exchange textual content, 4) present attainable completions (a function referred to as Intellisense or Autocompletion in different IDEs), 5) change the font kind and measurement, and rather more.
On high of this, you should utilize IDLE to create desktop functions.
Since we won’t be growing a desktop software on this 2-article sequence, be happy to decide on between the IDLE and the Python shell to observe the examples.
Do Primary Operations with Python on Linux
As is to be anticipated, you possibly can carry out arithmetic operations (be happy to make use of as many parentheses as wanted to carry out all of the operations you need!) and manipulate textual content strings very simply with Python.
You too can assign the outcomes of operations to variables and show them on the display screen. A useful function in Python is concatenation – simply provide the values of variables and/or strings in a comma-delimited record (inside parentheses) to the print perform and it’ll return the sentence composed by the objects within the sequence:
>>> a = 5 >>> b = 8 >>> x = b / a >>> x 1.6 >>> print(b, "divided by", a, "equals", x)
Word which you can combine variables of various varieties (numbers, strings, booleans, and so on) and after you have assigned a worth to a variable you possibly can change the information kind with out issues later (because of this Python is alleged to be a dynamically typed language).
Should you try to do that in a statically typed language (equivalent to Java or C#), an error will probably be thrown.
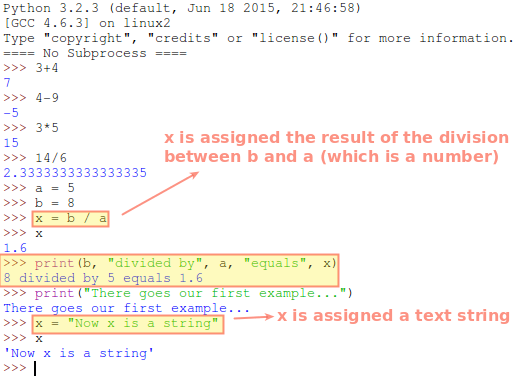
A Transient Remark About Object-Oriented Programming
In Object Oriented Programming (OOP), all entities in a program are represented as objects and thus they will work together with others. As such, they’ve properties and most of them can carry out actions (referred to as strategies).
For instance, let’s suppose we need to create a canine object. A few of the attainable properties are coloration, breed, age, and so on, whereas a number of the actions a canine can carry out are bark(), eat(), sleep(), and lots of others.
Strategies names, as you possibly can see, are adopted by a set of parentheses which can (or could not) include one (or extra) arguments (values which are handed to the strategy).
Let’s illustrate these ideas with one of many fundamental object varieties in Python: lists.
Illustrating Strategies and Properties of Objects: Lists in Python
An inventory is an ordered group of things, which don’t essentially should be the entire identical information varieties. To create an empty record named rockBands, use a pair of sq. brackets as follows:
To append an merchandise to the tip of the record, go the merchandise to the append()
technique as follows:
>>> rockBands = [] >>> rockBands.append("The Beatles") >>> rockBands.append("Pink Floyd") >>> rockBands.append("The Rolling Stones")
To take away an merchandise from the record, we will go the precise factor to the take away()
technique, or the place of the factor (rely begins at zero) within the record to pop()
.
In different phrases, we will use both of the next choices to take away “The Beatles” from the record:
>>> rockBands.take away("The Beatles") or >>> rockBands.pop(0)
You may show the record of accessible strategies for an object by urgent Ctrl + Area
when you’ve typed the identify adopted by a dot:
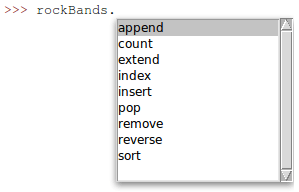
A property of an inventory object is the variety of objects it incorporates. It’s really known as size and is invoked by passing the record as an argument to the len built-in perform (by the best way, the print assertion, which we exemplified earlier-, is one other Python built-in perform).
Should you kind len adopted by opening parentheses within the IDLE, you will note the default syntax of the perform:
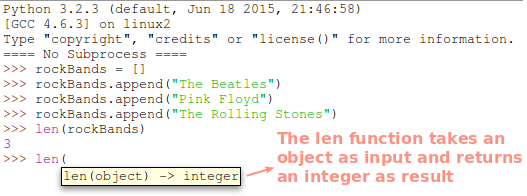
Now, what concerning the particular person objects on the record? Have they got strategies and properties as effectively? The reply is sure. For instance, you possibly can convert a string merchandise to uppercase and get the variety of characters it incorporates as follows:
>>> rockBands[0].higher() 'THE BEATLES' >>> len(rockBands[0]) 11
Abstract
On this article, we have now offered a short introduction to Python, its command-line shell, and the IDLE, and demonstrated the right way to carry out arithmetic calculations, the right way to retailer values in variables, the right way to print again these values to the display screen (both by itself or as a part of a concatenation), and defined by way of a sensible instance what are the strategies and properties of an object.
Within the subsequent article, we are going to focus on management circulation with conditionals and loops. We can even show the right way to use what we have now discovered to jot down a script to assist us in our sysadmin duties.
Does Python sound like one thing you want to be taught extra about? Keep tuned for the second half on this sequence (the place amongst different issues we are going to mix the bounties of Python and command-line instruments in a script), and likewise take into account shopping for the finest udemy python programs to improve your data.
As at all times, you possibly can rely on us when you’ve got any questions on this text. Simply ship us a message utilizing the contact kind beneath and we are going to get again to you as quickly as attainable.