Within the first chapter of the collection, I shared my ideas on why Rust is an more and more fashionable programming language. I additionally confirmed find out how to write Good day World program in Rust.
Let’s proceed this Rust journey. On this article, I shall introduce you to variables and constants within the Rust programming language.
On high of that, I can even cowl a brand new programming idea known as “shadowing”.
The individuality of Rust’s variables
A variable within the context of a programming language (like Rust) is called an alias to the reminiscence tackle by which some knowledge is saved.
That is true for the Rust programming language too. However Rust has one distinctive “function”. Each variable that you just declare is immutable by default. Which means as soon as a price is assigned to the variable, it can’t be modified.
This resolution was made to make sure that, by default, you do not have to make particular provisions like spin locks or mutexes to introduce multi-threading. Rust ensures secure concurrency. Since all variables (by default) are immutable, you don’t want to fret a couple of thread altering a price unknowingly.
This isn’t to say that variables in Rust are like constants as a result of they don’t seem to be. Variables might be explicitly outlined to permit mutation. Such a variable known as a mutable variable.
Following is the syntax to declare a variable in Rust:
// immutability by default
// the initialized worth is the **solely** worth
let variable_name = worth;
// mutable variable outlined by way of 'mut' key phrase
// the preliminary worth might be modified to one thing else
let mut variable_name = worth;
🚧
Which means, when you have a mutable variable of sort float, you cannot assign a personality to it down the highway.
Excessive-level overview of Rust’s knowledge sorts
Within the earlier article, you might need seen that I discussed that Rust is a strongly typed language. However to outline a variable, you do not specify the information sort, as an alternative, you employ a generic key phrase let
.
The Rust compiler can infer the information sort of a variable based mostly on the worth assigned to it. However it may be accomplished if you happen to nonetheless want to be express with knowledge sorts and need to annotate the kind. Following is the syntax:
let variable_name: data_type = worth;
A number of the widespread knowledge sorts within the Rust programming language are as follows:
- Integer sort:
i32
andu32
for signed and unsigned, 32-bit integers, respectively - Floating level sort:
f32
andf64
, 32-bit and 64-bit floating level numbers - Boolean sort:
bool
- Character sort:
char
I’ll cowl Rust’s knowledge sorts in additional element within the subsequent article. For now, this ought to be ample.
🚧
Rust doesn’t have implicit typecasting. So if you happen to assign the worth 8
to a variable with a floating level knowledge sort, you’ll face a compile time error. What it’s best to assign as an alternative is the worth 8.
or 8.0
.
Rust additionally enforces {that a} variable be initialized earlier than the worth saved in it’s learn.
{ // this block will not compile
let a;
println!("{}", a); // error on this line
// studying the worth of an **uninitialized** variable is a compile-time error
}
{ // this block will compile
let a;
a = 128;
println!("{}", a); // no error right here
// variable 'a' has an preliminary worth
}
Should you declare a variable with out an preliminary worth and use it earlier than assigning it some preliminary worth, the Rust compiler will throw a compile time error.
Although errors are annoying. On this case, the Rust compiler is forcing you to not make one of many quite common errors one makes when writing code: un-initialized variables.
Rust compiler’s error messages
Let’s write a couple of packages the place you
- Perceive Rust’s design by performing “regular” duties, which are literally a significant reason behind memory-related points
- Learn and perceive the Rust compiler’s error/warning messages
Testing variable immutability
Allow us to intentionally write a program that tries to change a mutable variable and see what occurs subsequent.
fn essential() {
let mut a = 172;
let b = 273;
println!("a: {a}, b: {b}");
a = 380;
b = 420;
println!("a: {}, b: {}", a, b);
}
Appears to be like like a easy program to this point till line 4. However on line 7, the variable b
–an immutable variable–gets its worth modified.
Discover the 2 strategies of printing the values of variables in Rust. On line 4, I enclosed the variables between curly brackets in order that their values can be printed. On line 8, I hold the brackets empty and supply the variables as arguments, C fashion. Each approaches are legitimate. (Aside from modifying the immutable variable’s worth, everyting on this program is right.)
Let’s compile! You already understand how to try this if you happen to adopted the earlier chapter.
$ rustc essential.rs
error[E0384]: can't assign twice to immutable variable `b`
--> essential.rs:7:5
|
3 | let b = 273;
| -
| |
| first task to `b`
| assist: contemplate making this binding mutable: `mut b`
...
7 | b = 420;
| ^^^^^^^ can't assign twice to immutable variable
error: aborting because of earlier error
For extra details about this error, attempt `rustc --explain E0384`.
📋
The phrase ‘binding’ refers back to the variable title. That is an oversimplification, although.
This completely demonstrates Rust’s sturdy error checking and informative error messages. The primary line reads out the error message that forestalls the compilation of the above code:
error[E0384]: can't assign twice to immutable variable b
It signifies that the Rust compiler seen that I used to be attempting to re-assign a brand new worth to the variable b
however the variable b
is an immutable variable. So that’s inflicting this error.
The compiler even identifies the precise line and column numbers the place this error is discovered.
Beneath the road that claims first task to `b`
is the road that gives assist. Since I’m mutating the worth of the immutable variable b
, I’m informed to declare the variable b
as a mutable variable utilizing the mut
key phrase.
🖥️
Implement a repair by yourself to raised perceive the issue at hand.
Enjoying with uninitialized variables
Now, let us take a look at what the Rust compiler does when an uninitialized variable’s worth is learn.
fn essential() {
let a: i32;
a = 123;
println!("a: {a}");
let b: i32;
println!("b: {b}");
b = 123;
}
Right here, I’ve two immutable variables a
and b
and each are uninitialized on the time of declaration. The variable a
will get a price assigned earlier than its worth is learn. However the variable b
‘s worth is learn earlier than it’s assigned an preliminary worth.
Let’s compile and see the consequence.
$ rustc essential.rs
warning: worth assigned to `b` is rarely learn
--> essential.rs:8:5
|
8 | b = 123;
| ^
|
= assist: perhaps it's overwritten earlier than being learn?
= observe: `#[warn(unused_assignments)]` on by default
error[E0381]: used binding `b` is possibly-uninitialized
--> essential.rs:7:19
|
6 | let b: i32;
| - binding declared right here however left uninitialized
7 | println!("b: {b}");
| ^ `b` used right here however it's possibly-uninitialized
|
= observe: this error originates within the macro `$crate::format_args_nl` which comes from the growth of the macro `println` (in Nightly builds, run with -Z macro-backtrace for more information)
error: aborting because of earlier error; 1 warning emitted
For extra details about this error, attempt `rustc --explain E0381`.
Right here, the Rust compiler throws a compile time error and a warning. The warning says that the variable b
‘s worth is rarely being learn.
However that is preposterous! The worth of variable b
is being accessed on line 7. However look intently; the warning is relating to line 8. That is complicated; let’s briefly skip this warning and transfer on to the error.
The error message reads that used binding `b` is possibly-uninitialized
. Like within the earlier instance, the Rust compiler is mentioning that the error is brought on by studying the worth of the variable b
on line 7. The explanation why studying the worth of the variable b
is an error is that its worth is uninitialized. Within the Rust programming language, that’s unlawful. Therefore the compile time error.
🖥️
This error might be simply solved by swapping the codes of strains 7 and eight. Do it and see if the error goes away.
Instance program: Swap numbers
Now that you’re accustomed to the widespread variable-related points, let us take a look at a program that swaps the values of two variables.
fn essential() {
let mut a = 7186932;
let mut b = 1276561;
println!("a: {a}, b: {b}");
// swap the values
let temp = a;
a = b;
b = temp;
println!("a: {}, b: {}", a, b);
}
Right here, I’ve declared two variables, a
and b
. Each variables are mutable as a result of I want to change their values down the highway. I assigned some random values. Initially, I print the values of those variables.
Then, on line 8, I create an immutable variable known as temp
and assign it the worth saved in a
. The explanation why this variable is immutable is as a result of temp
‘s worth is not going to be modified.
To swap values, I assign the worth of variable b
to variable a
and on the subsequent line I assign the worth of temp
(which comprises worth of a
) to variable b
. Now that the values are swapped, I print values of variables a
and b
.
When the above code is compiled and executed, I get the next output:
a: 7186932, b: 1276561
a: 1276561, b: 7186932
As you may see, the values are swapped. Excellent.
Utilizing Unused variables
When you could have declared some variables you propose to make use of down the road however haven’t used them but, and compile your Rust code to verify one thing, the Rust compiler will warn you about it.
The explanation for that is apparent. Variables that won’t be used take up pointless initialization time (CPU cycle) and reminiscence house. If it is not going to be used, why have it in your program within the first place?
However typically, you is perhaps in a scenario the place making a variable may not be in your palms. Say when a perform returns a couple of worth and also you solely want a couple of values. In that case, you may’t inform the library maintainer to regulate their perform in accordance with your wants.
So, in instances like that, you may have a variable that begins with an underscore and the Rust compiler will now not provide you with such warnings. And if you happen to actually don’t have to even use the worth saved in stated unused variable, you may merely title it _
(underscore) and the Rust compiler will ignore it too!
The next program is not going to solely not generate any output, however it’s going to additionally not generate any warnings and/or error messages:
fn essential() {
let _unnecessary_var = 0; // no warnings
let _ = 0.0; // ignored fully
}
Arithmetic operations
Since math is math, Rust would not innovate on it. You need to use the entire arithmetic operators you might need utilized in different programming languages like C, C++ and/or Java.
A whole record of all of the operations within the Rust programming language, together with their that means, might be discovered right here.
Instance Program: A Rusty thermometer
Following is a typical program that converts Fahrenheit to Celsius and vice a versa.
fn essential() {
let boiling_water_f: f64 = 212.0;
let frozen_water_c: f64 = 0.0;
let boiling_water_c = (boiling_water_f - 32.0) * (5.0 / 9.0);
let frozen_water_f = (frozen_water_c * (9.0 / 5.0)) + 32.0;
println!(
"Water begins boiling at {}°C (or {}°F).",
boiling_water_c, boiling_water_f
);
println!(
"Water begins freezing at {}°C (or {}°F).",
frozen_water_c, frozen_water_f
);
}
Not a lot is occurring right here… The Fahrenheit temperature is transformed to Celsius and vice a versa for the temperature in Celsius.
As you may see right here, since Rust doesn’t permit computerized sort casting, I needed to introduce a decimal level to the entire numbers 32, 9 and 5. Aside from that, that is just like what you’d do in C, C++ and/or Java.
As a studying train, attempt writing a program that finds out what number of digits are in a given quantity.
Constants
With some programming information, you may know what this implies. A relentless is a particular sort of variable whose worth by no means modifications. It stays fixed.
Within the Rust programming language, a continuing is asserted utilizing the next syntax:
const CONSTANT_NAME: data_type = worth;
As you may see, the syntax to declare a continuing is similar to what we noticed in declaring a variable in Rust. There are two variations although:
- A relentless title ought to be in
SCREAMING_SNAKE_CASE
. All uppercase characters and phrases separated by an undercase. - Annotating the information sort of the fixed is needed.
Variables vs Constants
You is perhaps questioning, for the reason that variables are immutable by default, why would the language additionally embody constants?
The next desk ought to assist alleviate your doubts. (In case you are curious and need to higher perceive these variations, you may take a look at my weblog which reveals these variations intimately.)
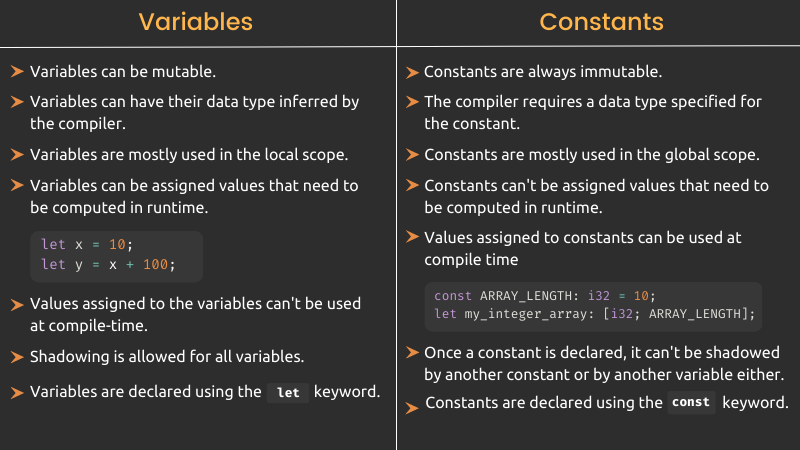
Instance program utilizing constants: Calculate space of circle
Following is an easy program about constants in Rust. It calculates the world and the perimeter of a circle.
fn essential() {
const PI: f64 = 3.14;
let radius: f64 = 50.0;
let circle_area = PI * (radius * radius);
let circle_perimeter = 2.0 * PI * radius;
println!("There's a circle with the radius of {radius} centimetres.");
println!("Its space is {} centimetre sq..", circle_area);
println!(
"And it has circumference of {} centimetres.",
circle_perimeter
);
}
And upon working the code, the next output is produced:
There's a circle with the radius of fifty centimetres.
Its space is 7850 centimetre sq..
And it has circumference of 314 centimetres.
Variable shadowing in Rust
In case you are a C++ programmer, you already form of know what I’m referring to. When the programmer declares a brand new variable with the identical title as an already declared variable, it is called variable shadowing.
In contrast to C++, Rust permits you to carry out variable shadowing in the identical scope too!
💡
When a programmer shadows an present variable, the brand new variable is assigned a brand new reminiscence tackle however is referred with the identical title as the present variable.
Allow us to check out the way it works in Rust.
fn essential() {
let a = 108;
println!("addr of a: {:p}, worth of a: {a}", &a);
let a = 56;
println!("addr of a: {:p}, worth of a: {a} // put up shadowing", &a);
let mut b = 82;
println!("naddr of b: {:p}, worth of b: {b}", &b);
let mut b = 120;
println!("addr of b: {:p}, worth of b: {b} // put up shadowing", &b);
let mut c = 18;
println!("naddr of c: {:p}, worth of c: {c}", &b);
c = 29;
println!("addr of c: {:p}, worth of c: {c} // put up shadowing", &b);
}
The :p
inside curly brackets within the println
assertion is just like utilizing %p
in C. It specifies that the worth is within the format of a reminiscence tackle (pointer).
I take 3 variables right here. Variable a
is immutable and is shadowed on line 4. Variable b
is mutable and can be shadowed on line 9. Variable c
is mutable however on line 14, solely it is worth is mutated. It’s not shadowed.
Now, let us take a look at the output.
addr of a: 0x7ffe954bf614, worth of a: 108
addr of a: 0x7ffe954bf674, worth of a: 56 // put up shadowing
addr of b: 0x7ffe954bf6d4, worth of b: 82
addr of b: 0x7ffe954bf734, worth of b: 120 // put up shadowing
addr of c: 0x7ffe954bf734, worth of c: 18
addr of c: 0x7ffe954bf734, worth of c: 29 // put up shadowing
Trying on the output, you may see that not solely the values of all three variables have modified, however the addresses of variables that had been shadowed are are additionally totally different (verify the previous couple of hex characters).
The reminiscence tackle for the variables a
and b
modified. Which means mutability, or lack thereof, of a variable is just not a restriction when shadowing a variable.
Conclusion
This text covers variables and constants within the Rust programming language. Arithmetic operations are additionally coated.
As a recap:
- Variables in Rust are immutable by default however mutability might be launched.
- Programmer must explicitly specify variable mutability.
- Constants are all the time immutable it doesn’t matter what and require sort annotation.
- Variable shadowing is declaring a new variable with the identical title as an present variable.
Superior! Good going with Rust i imagine. Within the subsequent chapter, I am going to focus on Knowledge Sorts in Rust. Keep Tuned.
In the meantime, when you have any questions, please let me know.